Neo4j: keep/filter keys in a map using APOC
In this post we’ll learn how to write a Cypher query to create a node in Neo4j containing some of the keys from a map. This post assumes that the APOC library is installed.
We’ll start by creating a map that contains data from my twitter profile:
:param document => {
id: 14707949, name: "Mark Needham",
username: "markhneedham", bio: "Developer Relations @neo4j",
location: "London, United Kingdom", url: "http://www.markhneedham.com",
join_date: "8 May 2008", join_time: "5:58 PM",
tweets: 24710, following: 2479, followers: 5054, likes: 1014
};
We want to create a User
node based on this data, but we don’t want to use all of the keys in the map.
If we want to remove some keys we can use the apoc.map.clean
function.
The following function call removes the join_date
and join_time
keys:
WITH ["join_date", "join_time"] AS keysToRemove
RETURN apoc.map.clean($document, keysToRemove, []) AS result
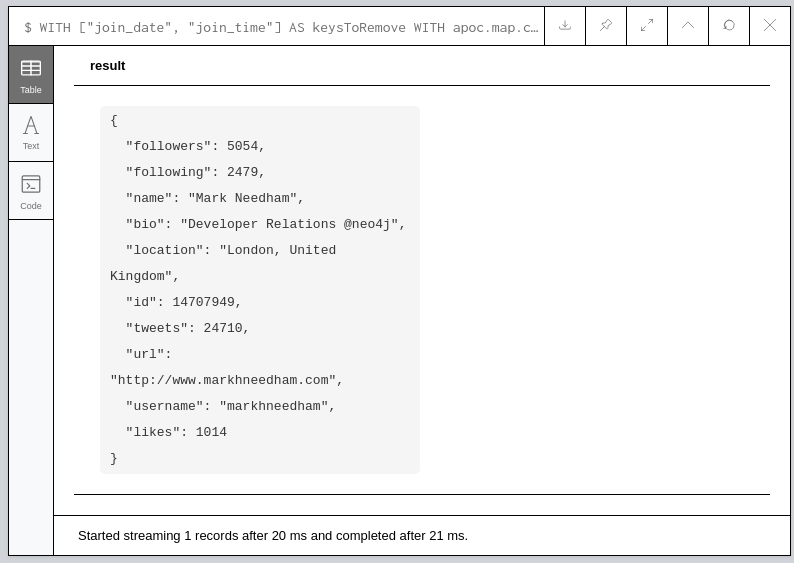
But what if we want to keep keys rather than remove them?
I ran apoc.help('apoc.map')
to see if there were any functions to do this, and as far as I can tell there aren’t:
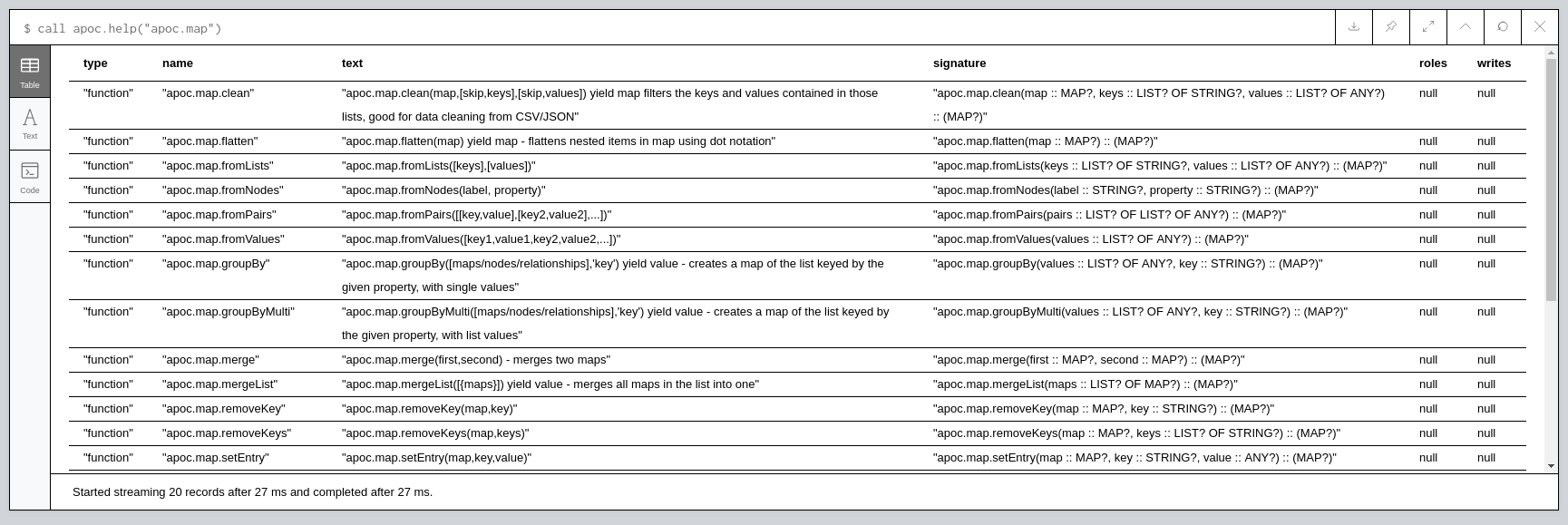
We can, however, combine two APOC functions to get the desired result.
First up, apoc.map.values
extracts the values for a list of keys:
WITH ["name", "username", "bio", "following", "followers"] AS keysToKeep
RETURN apoc.map.values($document, keysToKeep)
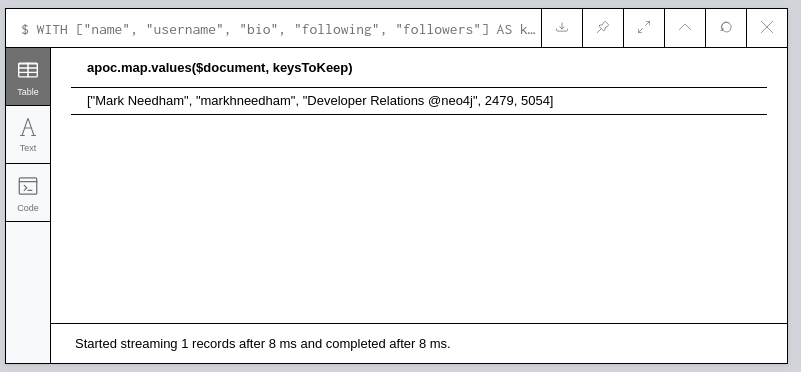
And we can then use apoc.map.fromLists
to reconstruct our map:
WITH ["name", "username", "bio", "following", "followers"] AS keysToKeep
RETURN apoc.map.fromLists(keysToKeep, apoc.map.values($document, keysToKeep)) AS result
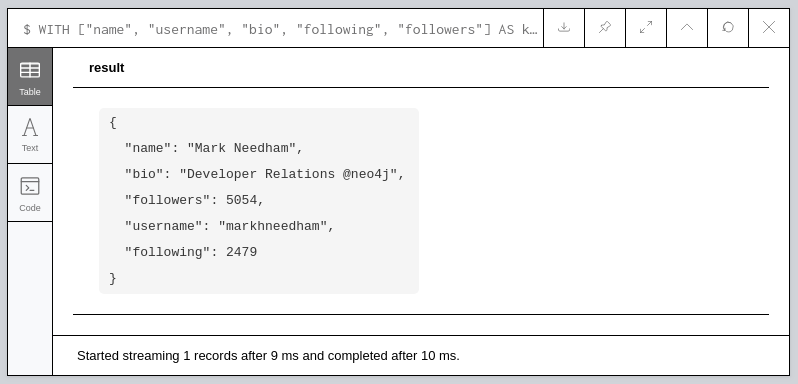
This code forms part of the following query that creates a User
node keyed on the id
property, and then sets the other properties afterwards:
WITH ["name", "username", "bio", "following", "followers"] AS keysToKeep
MERGE (u:User {id: $document.id })
SET u += apoc.map.fromLists($keysToKeep, apoc.map.values($document, $keysToKeep))
All done!
About the author
I'm currently working on short form content at ClickHouse. I publish short 5 minute videos showing how to solve data problems on YouTube @LearnDataWithMark. I previously worked on graph analytics at Neo4j, where I also co-authored the O'Reilly Graph Algorithms Book with Amy Hodler.